Node.js has gained significant traction in recent years as a powerful JavaScript runtime environment. Built on Chrome's V8 engine, it offers speed and efficiency, making it a preferred choice for modern web applications.
Getting started with a Node.js project is straightforward. However, as the application scales, maintaining a well-structured codebase and handling potential pitfalls become challenging. Without the right development practices, minor issues can escalate, impacting performance and stability.
To bridge the gap between a well-optimized production-ready application and a project riddled with inefficiencies, our Node.js developers follow 27 essential development practices. Let’s explore them in detail.
What is Node.js?
Released in 2009 by Ryan Dahl, Node.js has rooted itself deep in the world of web application development. Because of its higher consistency and better output, it is used for developing large-scale applications, such as single-page apps, video streaming platforms, and other web applications.
Node.js is an impeccable and robust framework developed on Chrome’s V8 Javascript engine that is one of the lightning-fast Javascript available in the markets.
It compiles JavaScript code right into the native machine code that will eventually boost an app’s efficiency. It extends JavaScript API for offering basic server-side functionalities.
Node.js practices for effective development
With that said, let’s have a look at the best Node.js practices that will rescue you from falling on common Node.js traps when you plan to hire dedicated developers:
1. Start all projects with npm init
NPM is the best way of adding couplings or dependencies. However, the usage of npm is not just limited to these two things, you can use it to explore the entire Node.js packages.
Create a new project in Node.js using npm init
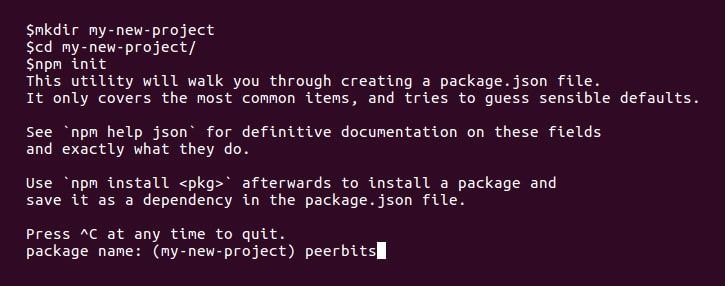
Doing so will create a new package.json and you can add a bunch of metadata, which will help others on the same project.
Besides this, you can add an updated Node.js version within the package that follows the semantic versioning spec.
2. Setup .npmrc
The .npmrc file assures npm install always updates the package.json and imposes the version of installed dependency to be an exact match.
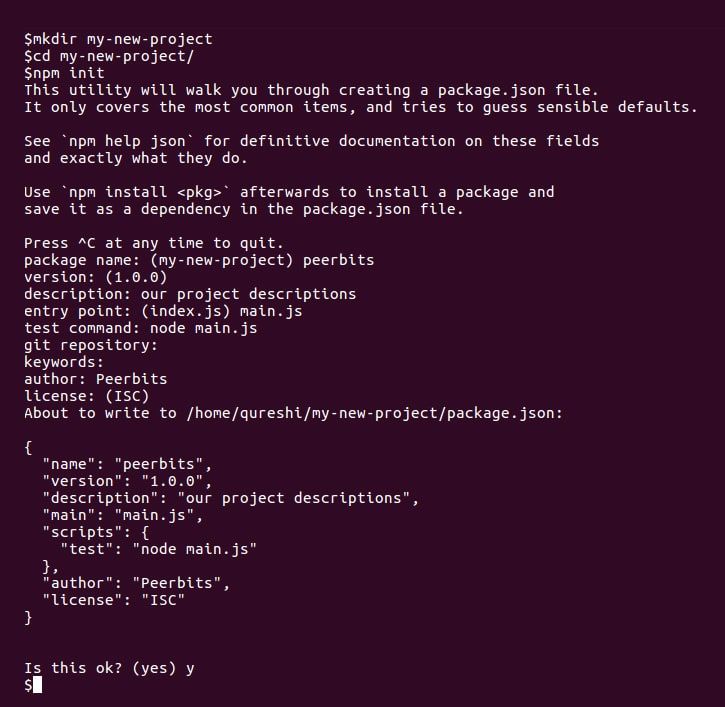
Add a scripts property and object to your package.json with a start key.
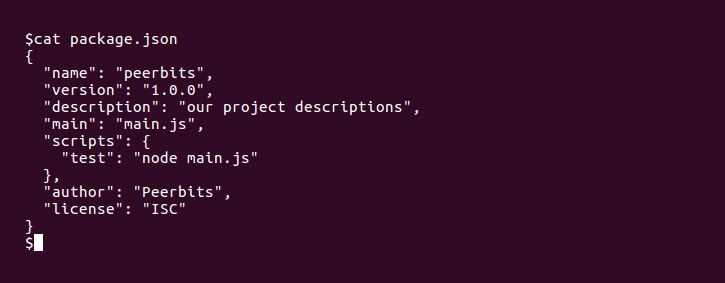
Now, whenever somebody will run npm start, npm will node myapp.js along with all the dependencies from node_modules/.bin on the $PATH and you don’t have to global installs of NPM.
3. Use environment variables
Configuration management is an essential part of every kind of programming language. This part mainly deals with the procedure of decoupling the code, services, databases, etc, during development, production, or during quality analysis.
Use environment variables in Node.js to look up the values from process.env in your code. You can check the NODE_ENV environment variables.
The Node JS Best Practices will utilize the environment variable in your coding at this point. It would be utilised to find the values in the development process. nvm command.
A majority of cloud-hosting vendors use nvm to avoid confusion. Apart from this, you can use other consequent plans, regular expressions and configurations too.
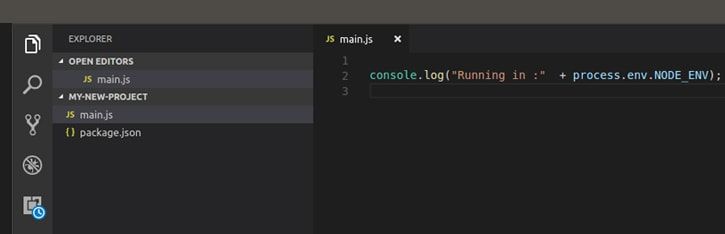
4. Use a style guide
Pick an existing set of guidelines for coding in Node.js and adhere to it to maintain code readability across the project chain.
However, if your style differs from the developer who had worked on a good number of Node.js projects previously then you may exchange the spaces with tabs or reformat the position of braces. This will take a good amount of your time and make the task boring for you.
Generally, the choice of style is subjective for the developers. So it is advisable for you to analyze the style guides used by your competitors to get a better idea. Here are some style guide used by the global tech giants:
Airbnb - https://github.com/airbnb/javascript
Google - https://google.github.io/styleguide/javascriptguide.xml
jQuery - https://contribute.jquery.org/style-guide/js/
5. Say no to synchronous functions
Synchronous functions block any other codes from executing until they have finished execution. To make sure no synchronous function is a part of the code, turn on -trace-sync-io flag and it will display a warning upon encountering asynchronous API.
Asynchronous API stops other codes to run while it is in action. Moreover, these functions are extremely important to simplify the logic flow of an application for other programmers as well.
In addition to this, embracing async functions in your coding would also notify you about your app’s asynchronous API. It automatically prints warning and stack traces at the time of the testing process.
6. Handle Errors
A small error can adversely affect your development process by bringing it entirely down is never a good experience. Accurate exception management is very important for an application. And the best way to deal with the different programmer errors is to implement the asynchronous code structures that are mentioned in this blog.
For instance, promises provide a .catch() handler that will circulate all errors to be dealt with, neatly.
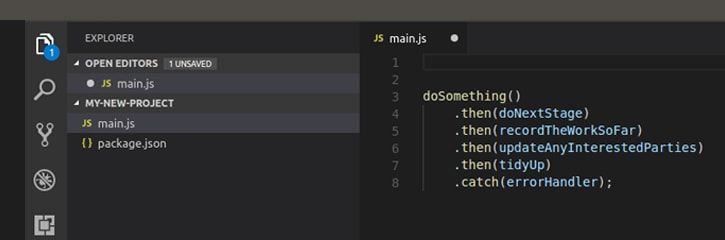
Regardless, which of the earlier functions could have failed, any operational error will result in the errorHandler.
7. Confirm your app automatically restarts
Even after following the premium error handling practices in development, some kind of dependency error may bring your app down. Use a process manager to ensure the app recuperates elegantly from a runtime error or when the server you’re running it on goes down.
Apart from this, there is another scenario where you need to restart your application. And that scenario is if the complete service that you are running gets down. In this case, you seek minimal time for your application to restart sooner. Also, you can use PM2 Keymetrics for managing your process.
8. Acquaint yourself with JavaScript best practices
Particularly, familiar yourself with best practices in writing asynchronous programming, scope, data types, function arguments, function & objects in JavaScript, and callbacks.
Along with this, you can take the help of Azure Functions or an Azure app service that can provide you with a serverless code infrastructure. This code infrastructure allows you to create responsive HTTP endpoints.
9. Cluster your app to improve reliability and performance
Because you want one process for each processing core so you can reallocate the capacity across all the CPU cores, to scalable web applications handling HTTP needs and presentation, one worker fails, the others are there to acknowledge requests.
Use cluster for flexibility, for the future, even when it is a single process on a tiny piece of hardware.
Read more: Create a chat app server using Node.js and Socket.io?
10. Entail all your dependencies up front
At all times, perform all your dependencies and organize them upfront. This way, you'll learn about a problem before it enthralls. Not after a customer finds it, a few hours your application has gone live.
And that is why you should always load your entire dependencies upfront and configure them accordingly. By doing this, you will know from the startup whether there is a problem with your application. Otherwise, your app will get live in production after some hours.
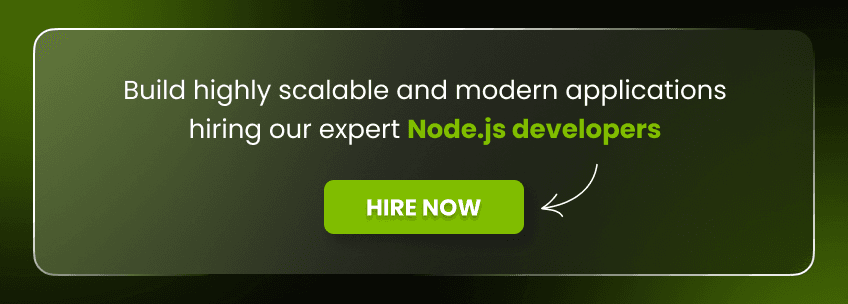
11. Use Gzip compression
The server can compress the response before sending it to a web browser with the help of Gzip compression and thus reduces time lag and latency.
12. Concatenating multiple JS files
Improve application performance is by shrinking and concatenating multiple JavaScript files. For example because a web browser will make six separate HTTP requests for each JavaScript files, which will create a block and raise wait time.
13. Employ client-side rendering
Employing client-side rendering in your Node.js project will considerable lessen latency and save bandwidth. Client-side JS frameworks—BackboneJS and AngularJS expose APIs which direct JSON responses, directly to the client, not via server where every request returns an HTML page.
14. Only git the significant bits
You should not track anything that's developed when using a source control system like git. It causes redundant bloat and chaos in your git history when you trail generated files for changes.
15. Application Monitoring
It is important to be notified when your application goes down, particularly in production apps. So, using something to monitor and notify you to serious issues or irregular behavior is vital.
16. Test your code
Whatsoever stage you are in a project, it’s never very late to introduce code quality testing. Start small, start simple.
17. Hop on the ES6 train
Node 4+ introduces an updated V8 engine with numerous valuable ES6 features Don’t be daunted by some of the extra compound stuff, you can acquire it as you go. There are adequate simple improvements for instant satisfaction.
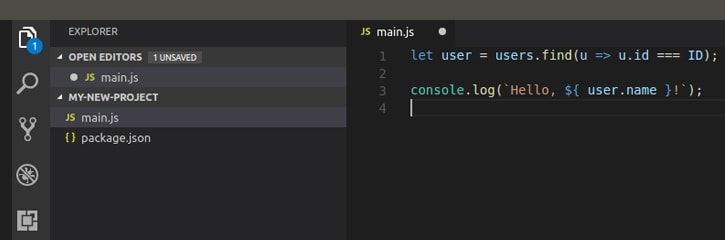
18. Stick to lowercase
Some programming languages inspire filenames that reflect class names, like MyClass and 'MyClass.js’. In Node.js, it is a complete no-no. As an alternative, use lowercase files:
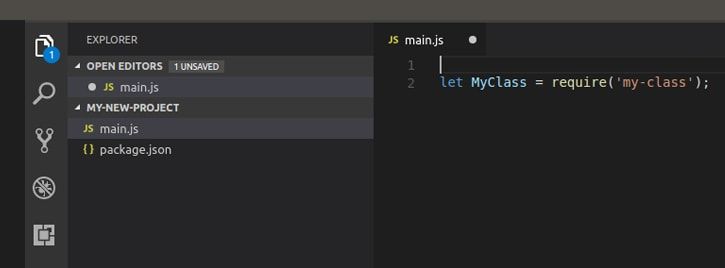
19. Avoid garbage
Node (V8) uses a idle and acquisitive garbage collector. It limit is 1.5 GB, it mostly delays until it has to reclaim unused memory. If your memory usage is swelling, it might not be a leak - but somewhat node’s typical idle behavior.
To regulate your app’s garbage collector, you can raise flags to V8 in your Procfile:

20. Use ES2015
ES2015 support has grown to 99% with Node v6.
21. Use Promises
Promises are a concurrency primitive and a part of most contemporary programming languages to make the life of developers easier.
22. Use Docker
Using a docker empowers your applications to run in isolation, makes your deployments securer. Docker images are lightweight, they allow absolute deployments, and with Node.js docker, you can reflect production settings in the vicinity. Apart from this, you can use the docker container for better performance.
23. Use Messaging for Background Processes
If you employ HTTP to send messages, then every time the receipt party is down, those messages are gone. Therefore, pick a persistent transport layer, like a message train to direct messages, you won't have to run in this issue.
24. Use the Latest LTS Node.js version
To receive the best of both worlds (stability and new features) we endorse using the newest LTS (long-term support) version of Node.js.

To switch Node.js version, use nvm and switch to LTS takes in two commands:
25. Use efficient tools to restart your app
Sometimes, you will have to stop and restart your application to apply even a simple change made to the code base in case if you are not using additional development tools. After coding for a while, this becomes a never-ending hustle.
But now you do not have to worry as you can use some core tools for professional developers to monitor an application’s codebase and restart the app automatically every time a change is made.
Some of the popular code monitoring packages available for Node are as follows:
Nodemon
Nodemon automatically restarts the application whenever a fresh change is made to the code. You can initialize Nodemon by replacing the node with nodemon on the command line.
Forever
Forever provides automatic restarting along with additional configuration options. These options include writing logs and setting a working directory that would normally be printed on stdout to a file.
PM2
PM2 is an excellent process management tool you can use to better up your development. It allows more control and features to manage processes running in production compared to the other two. Furthermore, it ensures that the application restarts as quickly as possible in case the server it’s running on goes down.
Security
If you want to combat any kind of attack, then you should think like an attacker or hacker first. As the amount of attack types is highly critical, developer security becomes essential. Having in-depth research of vulnerable code attack vectors will save you from malicious attacks.
Apart from this, you need to arrange a threat scrutinizing meeting every month. In this meeting, you and your front-end development team need to look at the app structure and suggest possible vulnerabilities or threats.
You can add some games or fun activities with this process so that it does not become boring for your front-end developers. Along with this, you can organize a competition between the team that designs the module and the read team that tries to exploit it.
26. ES Lint to optimize your code
There are numerous mistakes in Node JS development that can damage the total outcome of the process. However, there are some ways to combat this damage, such as async structures, that would be extremely helpful with error management.
ESLint is a type of code analysis that lets you analyze your code quickly to find problems. Generally, ESLint contains in-built text editors that you can run as part of a continuous integration pipeline.
To elaborate, while using a chain of this structure, one of them may fail without warning. You can sort out this error by using a suitable command line.
27. Run Parallel for optimizing speed
Developers need to assure a parallel flow of execution at the time of requesting remote services, file system access and database calls. By running many things at a single time will reduce latency and minimize the blocking operations.
Technically, Node.js does not execute multiple things at the same time. Instead, it pushes each task to an asynchronous event loop with no control of the task will finish before each other. If your execution needs to complete one or more tasks before the other, then consider going asynchronous.
Conclusion
Node.js remains a leading choice for web development due to its efficiency, scalability, and flexibility. Following the right development practices ensures smooth performance and long-term maintainability.
If you want to build a high-quality application, you can implement best practices yourself or hire dedicated Node.js developers who have the expertise to handle everything from architecture to deployment. With the right team, your project can achieve faster turnaround times and seamless execution.
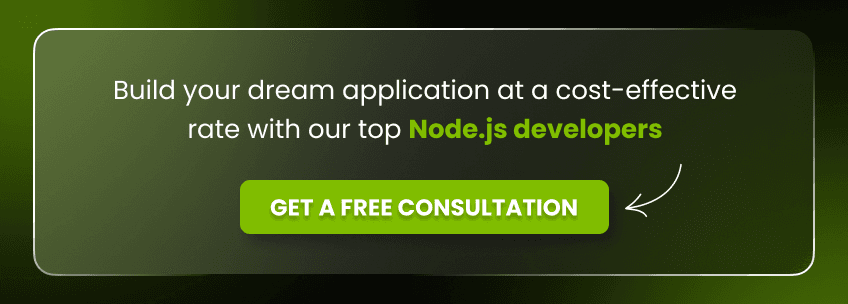