Testing your Angular app is a lot like double-checking your work before hitting "submit". You want to make sure that everything is just perfect - no typing mistakes and no missed attachments. But with Angular applications, testing isn't just about spotting small mistakes. It is about making sure that every part of your application works smoothly, from individual functions to entire user journeys.
Well, this blog will walk you through all the details about Angular unit testing, integration testing, and end-to-end (E2E) testing for applications. It also focuses on how tools like Jasmine, Karma, TestBed, Cypress & Protractor make the testing process a whole lot easier. Explore features, pros, and cons of each type of Angular testing to help you build a resilient post-production strategy for app development. Let’s take the ‘testing-drive’?
But before you directly get into specifics, Let’s just start with the basics.
Why you need testing in Angular apps?
Testing makes certain that your Angular app does not crash when users do something unexpected (it is very sure, they will). For that, you need to Hire Angular developers to build effective and efficient Angular applications.
Angular apps have a dynamic nature, which means bugs can easily sneak in during development. Proper testing helps prevent those nasty surprises.
-
Unit tests make sure that individual components are functioning,
-
Integration tests verify that everything works together, and
-
End-to-end tests check the overall app experience.
Now, let’s jump into each type of testing and how they help build more reliable Angular applications.
Unit testing with Angular
Unit testing is just like reviewing the smallest parts of your app. In Angular, you often test a single function, service, or component. Think of it as inspecting individual gears in a machine to just make sure that each of them works independently.
Here are some of the basic features of unit testing in Angular:
-
Isolated environment: Unit tests focus on a single and small part of your code at a time.
-
Speed: Since you’re testing small pieces usually unit tests are fast.
-
Early bug detection: Before issues grow into bigger problems you can check over them earlier in the development process.
Unit testing tools: Jasmine and Karma
In Angular, Jasmine is a framework that fits for writing unit tests. It provides an easy syntax for setting up test cases. With Karma, the tests can be automated and run across multiple browsers to make sure that they are compatible.
Well, a dedicated Angular developer needs to be skilled in utilizing these tools as they play a vital role in building high-quality applications with reliable functionality. Let’s get some detailed info about these testing tools of Angular:
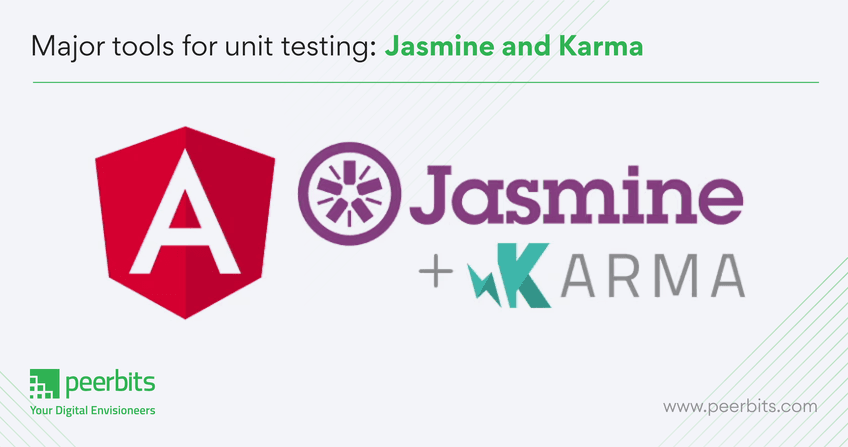
Jasmine is a popular testing framework used in Angular for writing unit and integration tests. It provides a clean and readable syntax that makes it easier to create test cases for individual components or services. With built-in matchers like toBe
and toEqual
, Jasmine just simplifies the process of validating expected outcomes in your application.
Core features:
-
Test setup: Jasmine's test suites (
describe
blocks) help you organise tests logically. -
Matchers: Jasmine provides built-in matchers like
toBe
andtoEqual
to assert expected behavior.
Karma:
Karma is a test runner which is designed to automate the testing process in Angular. It executes tests written with frameworks like Jasmine across multiple browsers, ensuring your app works consistently in different environments. Karma integrates well with continuous integration pipelines and also provides real-time feedback on test results.
Core features:
-
Test automation: Karma runs the tests automatically and reports results in real-time. Professional Angular developers for hire find it useful for automating repetitive tasks and verifying quality results.
-
Multi-browser support: Karma ensures your tests work in various browsers, avoiding unexpected cross-browser issues.
Advantages of unit testing:
-
Quick feedback: You get immediate insight into how well individual parts of your app are working.
-
Focused bug detection: It is easier to identify and fix issues when you know exactly what part of your Angular code is broken.
Disadvantages of unit testing:
-
Limited scope: Unit tests don’t cover how components interact with each other, so they might miss bugs that occur during integration.
-
Ongoing maintenance: As your app grows, keeping all your unit tests up to date can become time-consuming.
By now, you have read the basics of unit testing and how it handles individual components function properly. Next, let’s explore integration testing in Angularjs, where multiple components come together.
Integration testing with Angular
Once you are confident that your components work in isolation, the next step is to make certain that they work together. That’s where you will need integration testing. It is all about testing how different parts of your application interact.
These are some key features of integration testing:
-
Component interaction: Instead of testing isolated units the integration tests examine how components and services collaborate.
-
Mid-level complexity: These tests are more complex than unit tests but still are manageable compared to full end-to-end tests.
-
Data flow validation: Integration tests help confirm that data passes correctly between services and components.
Integration testing tools: Jasmine, Karma, and TestBed
Jasmine and Karma are still the key players in integration testing, but TestBed comes into the picture for configuring and initializing Angular modules and components like a Pro brother!
TestBed:
TestBed is an Angular testing tool that just simplifies the process of setting up and initializing environments for unit and integration tests. It lets developers create test modules that simulate real-world conditions by configuring components and services, while also supporting dependency injection. After this TestBed becomes necessary for testing interactions between different parts of an Angular application in a controlled environment.
Advantages of integration testing:
-
Tests real-world interactions: It checks how different parts of your app development cycle work together and covers more system than unit tests.
-
Component collaboration: Integration testing validates the relationships between components and services. It also verifies that they interact correctly.
Disadvantages of integration testing:
-
More time-consuming: Writing and executing integration tests takes longer time than unit tests.
-
Harder to debug: Since multiple components are involved, pinpointing the source of a bug can be a bit tough.
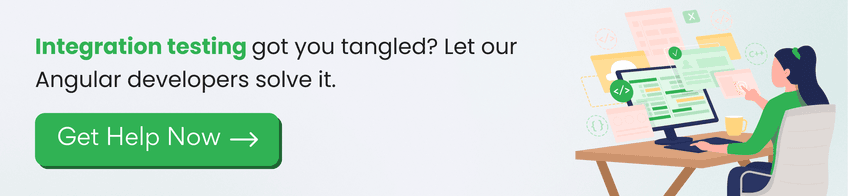
So far, you have checked individual components and tested how they interact. But there’s more to the story. You might be wondering- What happens when real users start interacting with the app? Exactly! that’s where end-to-end testing comes in.
End-to-end (E2E) testing with Angular
End-to-end testing is kind of playing the role of your user. It verifies that your app behaves well from the user’s perspective. It covers everything from the front-end to the back-end testing. If unit and integration tests check the parts, E2E tests make sure that the whole application model works smoothly.
Below are some of the key features of end-to-end testing:
-
Full application testing: E2E tests cover every aspect of your application, be it the front-end interface or the back-end services.
-
User behavior simulation: These tests mimic real user actions like clicking buttons, filling out forms, and navigating pages.
-
Cross-browser compatibility: E2E tests confirm your app functions correctly across various browsers and devices.
End-to-End testing tools: Protractor and Cypress
In Angular, Protractor has been the preferred tool for E2E testing, but Cypress is quickly gaining popularity due to its speed and ease of use. Let’s explore both Protractore and Cypress for E2E testing with Angularjs:
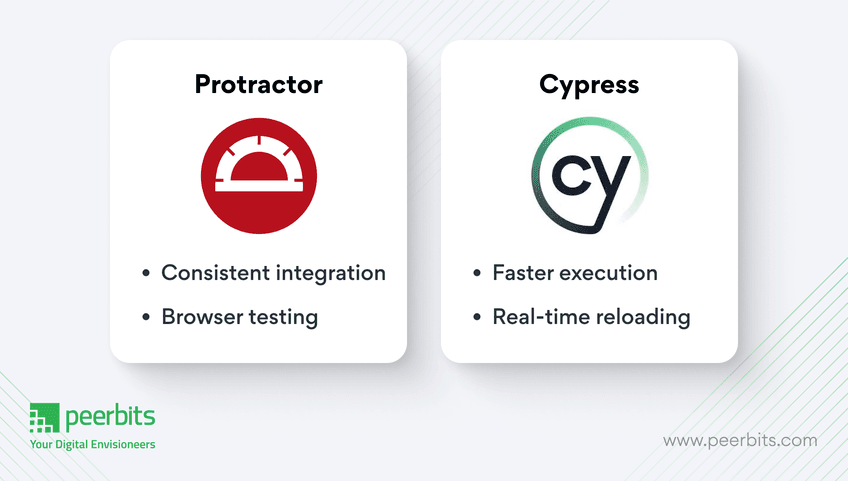
Protractor:
Protractor is an end-to-end testing framework specifically designed for Angular applications. It automates browser interactions to test the complete functionality of an app from the user’s perspective.
Protractor integrates with Angular’s specific features to handle asynchronous operations smoothly and verify that tests are synchronized with Angular’s execution cycle.
Consisten integration: Protractor integrates consistently with Angular and waits for the framework’s components to load before running tests.
Browser testing: It helps run E2E tests in multiple browsers to secure compatibility.
Cypress:
Now, Cypress is a modern End-to-End testing framework known for its speed and ease of use. It provides a simple setup and real-time reloading which makes writing and executing tests easier.
Cypress offers powerful debugging capabilities and a rich API for simulating user interactions. This makes it a very popular choice for testing Angular applications across different browsers and environments.
Faster execution: Cypress offers a simpler setup and faster execution, making it an attractive alternative to Protractor.
Real-time reloading: Cypress lets tests reload automatically when changes are made, speeding up the testing process.
Advantages of E2E testing:
-
Covers the entire app: E2E tests provide complete coverage. It also makes sure that your app works from start to finish.
-
Real-world bug detection: Since these tests simulate actual user behavior, they can catch issues that unit and integration tests might miss.
-
Cross-browser support: E2E tests help verify your app works correctly in different browsers, improving user experience across platforms.
Disadvantages of E2E testing:
-
Slow execution: Since E2E tests involve testing the entire app the process gets slower when compared to unit and integration tests.
-
Difficult debugging: When an E2E test fails, identifying the specific cause can be difficult due to its wide coverage.
-
High maintenance: With the growth of your app maintaining a suite of E2E tests can be a tough job.
For various top Angularjs development company doing E2E testing is a must to verify product quality across multiple platforms and devices.
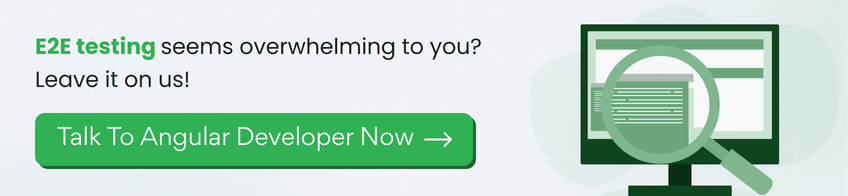
Balancing unit, integration & E2E testing with Angular
Each Angular testing type serves a different purpose, and they complement each other. Here’s a quick recap:
-
Unit testing checks the individual components.
-
Integration testing verifies how those components work together.
-
E2E testing confirms whether the entire app runs smoothly, mimicking real user behavior.
To build a reliable Angular app, you’ll need a balanced mix of all three. Unit testing catches small bugs early, integration testing ensures proper data flow, and E2E testing simulates the real user experience. Together, they create a strong testing strategy that keeps your app running smoothly. Whether you are aprofessional Angular dev or managing a larger team, a comprehensive approach to testing will help deliver successful projects.
To discover more details on Angular development—Explore a collection of expert blogs!
Creating Dynamic and Responsive Web Applications with AngularJS Development
Understanding the Costs: A Detailed Guide to Hiring a Dedicated Angular Developer
Angular Explained: Overview, History, Versions, Tools, Benefits, and Drawbacks
Conclusion
Testing your Angular app isn’t just a box to check off. It is a necessary part of delivering a reliable, user-friendly experience. Whether you are running unit tests on individual components, checking how your services interact with integration tests, or mimicking real-world scenarios with end-to-end tests, tools like Jasmine, Karma, TestBed, Protractor, and Cypress make it all possible. Hire Angular experts with expertise in testing, as they can make sure that your application meets user expectations and performs smoothly across all environments.
Incorporating a complete testing strategy into your Angular development process helps you catch potential issues and also builds confidence in your code. Whether it's isolating small units of functionality or simulating full user interactions, each type of test plays a critical role in confirming your app is strong and scalable. By making testing a priority, you are reducing the chances of post-release bugs and improving the general quality and performance of your application in the long run.
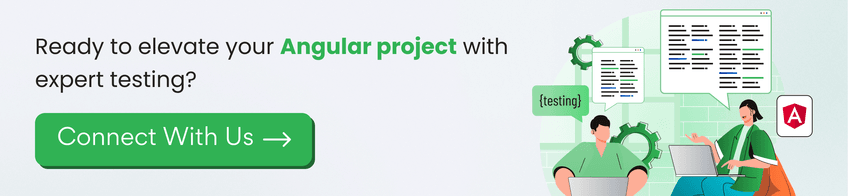