Major companies increasingly prioritize building scalable applications to handle growing user bases and complex data needs. A study by Gartner shows that businesses lose an average of $300,000 per hour due to application downtime, often caused by scalability issues. Event giants like Twitter faced massive outages in its early days due to scalability problems, leading to significant reputational damage. This makes scalability essential for both operational efficiency and business growth.
One of the effective solutions for achieving this is Python asynchronous programming. It is valued for its ability to manage thousands of simultaneous tasks without overloading system resources. It helps in processing large volumes of data, making it ideal for applications that require horizontal scaling.
Businesses prefer to adopt asynchronous Python frameworks to keep their applications responsive during peak hours and avoid the costly pitfalls experienced by other companies. Python tools like asyncio and coroutines help a team of Python developers create faster, more scalable applications by managing multiple tasks like Pros! Considering the high demand for scalability in this era and the risk of costly downtime, async programming is a key approach to staying competitive.
In this blog, you’ll explore Python’s asynchronous programming and its role in building scalable applications. Discover how non-blocking operations and tools like asyncio
improve efficiency, and get insights into the techniques that prevent scalability issues, with real-world examples. First, let’s get an overview of Async coding!
What is Asynchronous programming?
Asynchronous programming refers to the act of running more than one task at a time and continuing execution without waiting for the other tasks to finish. Think of it as watching pasta’s water boil while chopping veggies and stirring the sauce(Only multitasking pros can slay it!).
Additionally, testAsync
is non-blocking, and asynchronous programming does not make your code wait. Instead of blocking future steps, pipelines work, allowing tasks to proceed concurrently. In essence, while working with asynchronous operations, you'll often hear about non-blocking calls and how they improve system performance.
Further, you should know the concept of non-blocking operations and how it plays a critical role in improving system performance by allowing tasks to run concurrently without causing delays.
Non-blocking operations
Non-blocking behavior is one of the primary concerns for developers in pursuing asynchronous programming. By using non-blocking operations, your program does not have to pause and wait for one operation (task) to complete before moving on to the core task. Eventually, it makes your code faster, and more efficient and has more interactions in less time. Let’s explore the incredible tools of Python async programming, starting with Coroutines.
What are Python Coroutines?
Coroutines are like the superheroes of async programming in Python. They are what you call a generator function, which is like a sub-function that can pause and resume execution. Imagine a Netflix series where you pause the show, grab some snacks, and return knowing that you can resume play exactly where it finished.
Coroutines also give you an effective way to deal with I/O-bound processes in a non-blocking manner. This has been one of the pillars of asynchronous programming in Python and their numbers have exploded with satisfying methods appearing all over since Asyncio came along. Let's break down its functionality further!
How do Coroutines work?
In Python, a coroutine is defined with an async def. For example:
async def fetch_data():
print("Fetching data...")
await asyncio.sleep(2) Simulate a delay
print("Data fetched")
The await
keyword is very important cause it will stop that coroutine for the amount of time required to do an API call. Synchronous flow makes async programming super efficient for operations that take a long time, for eg. network requests or files I/O.
Rather than having to wait for a single source to respond before going on to the next one, you can get them all at once. You should use coroutines in cases when you need to fetch data from different sources simultaneously.
Example: Fetching data concurrently with asyncio
By far you know how coroutines operate on a fundamental level. Now the next step is to cover asyncio. If coroutines are the superheroes, then asyncio is the city of their guardianship. It offers the methods to run and collect coroutines and respond to events through event cut-points along with preventing tasks from becoming blocked in each other.
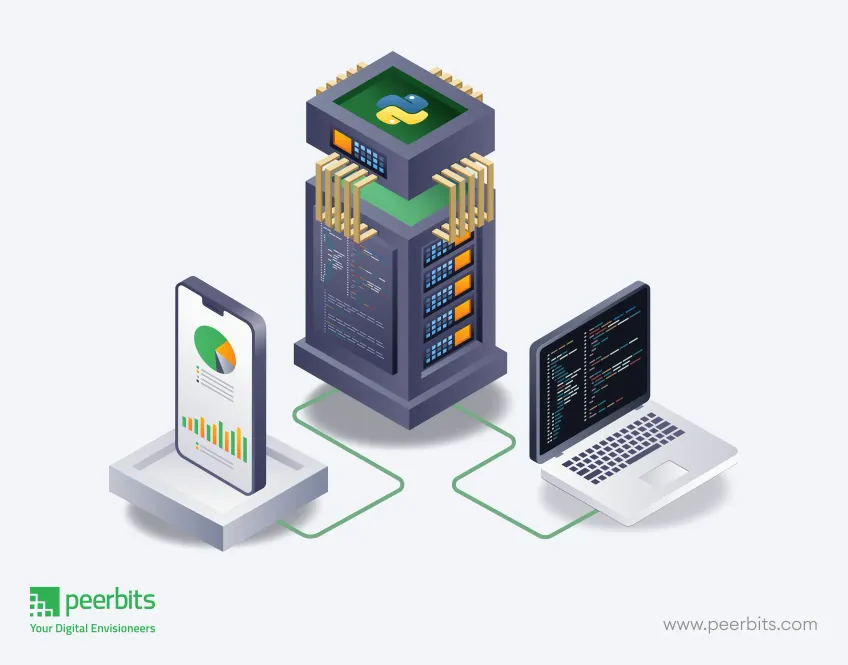
We have the event loop at the centre of asyncio. This is where the magic happens. The event loop is there to watch all of the tasks (coroutines) that you have running and switch between them as necessary. The cool part? All of this is done without you having to manage threads or processes manually.
For your ease, below is a simple example of using the event loop in code:
import asyncio
async def greet():
await asyncio.sleep(1)
print("Hello, Async World!")
async def main():
task1 = asyncio.create_task(greet())
task2 = asyncio.create_task(greet())
await task1
await task2
asyncio.run(main())
How async Coroutine help in scalability?
If you look around for the talk of Python async coroutines and scalability. It is because coroutines allow you to perform operations without blocking and makes the implementation of some applications possessing numerous I/O operations easier.
When to use coroutines?
If your app has to make thousands of requests or read a million files, coroutines will shine for Multiple I/O-bound Tasks.
Do you remember the times an app might not respond for a second while it was loading something? That's something async coroutines guarantee. It is more about making things work without tying up resources on wait. That is the reason you should always use coroutines to write scalable, non-blocking applications. Coroutines make both tasks run in parallel, and the event loop switches between them to make everything seamless.
Benefits of asynchronous programming
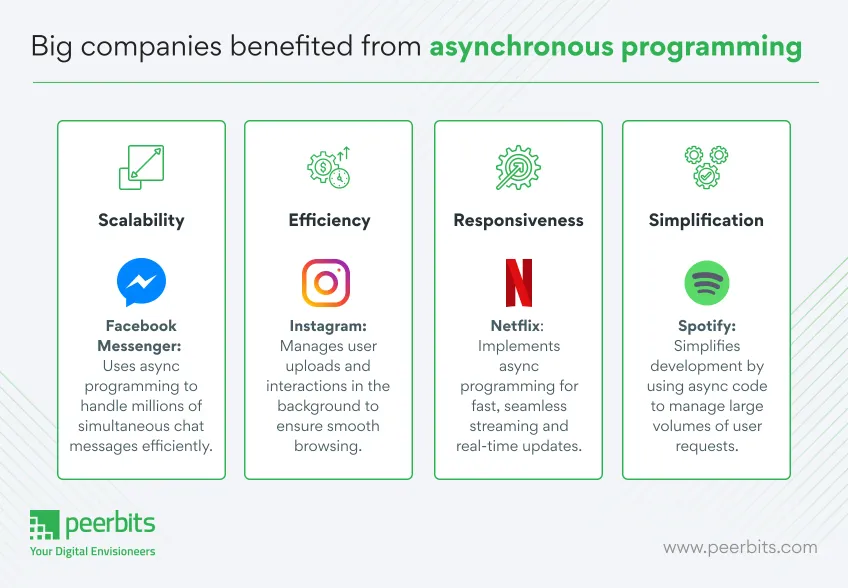
Discover the benefits of asynchronous programming, which allows applications to handle multiple tasks efficiently without delays. In this section, you will explore how non-blocking operations boost performance and scalability, with real-life examples that highlight the practical advantages.
1. Scalability
Async code allows you to run multiple tasks at a time. Especially if you are handling huge network communication or database queries, Async app can scale much better than others.
Example: Facebook Messenger deals with an enormous amount of real-time chat messages pouring in every second. With the help of asynchronous programming, they managed to excel flawlessly. Now Facebook Messenger can easily support millions of active connections, and no latencies are experienced. They are also able to scale well with async operations where it would be too difficult before, due to over-saturated servers.
2. Efficiency
Based on the execution model where no task is left for another to complete before jumping into action, it reduces overall time taken. Your system resources are utilized better.
Example: Instagram is an application of Meta, which uses networking at a higher level and allows them to scale efficiently their user upload, photo view & likes, and comment flow by using asynchronous programming. The processing takes place in the background when images or videos are uploaded so that your app is free to continue functionality.
3. Responsiveness
It means that your applications will not get freeze or stuck waiting for a slow task to finish. This is critical for web servers, which are required to handle several user requests in parallel.
Example: Netflix runs this type of web application where it uses asynchronous programming to keep its streaming service responsive. When the user chooses a video, multiple background operations are performed — metadata is loaded, and subtitles or suggestions appear from behind; all without slowing down playback. With this, users can watch a video in real time with zero buffering and lagging.
4. Simplification
Writing code that utilizes async is easier than working with multiple threads in Python. Further businesses who hire Python app developers also don’t have to hassle for thread synchronization and the risk of deadlocks.
Example: Spotify (a giant music company) struggled with traditional multi-threading and process management during peak usage, leading to crashes. By switching to asynchronous programming, Spotify simplified development and ensured stable, responsive performance even with high user activity.
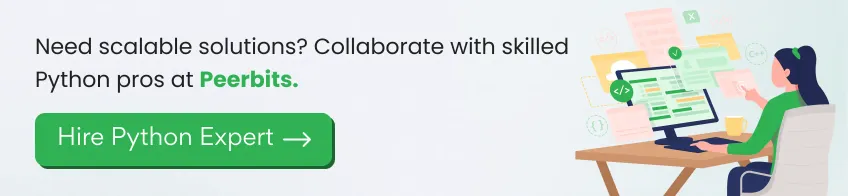
Hazards of async code
Surely, no technology is perfect, that’s why here are some of the challenges in async programming that can impact your app development processes:
1. Debugging is tougher
With asynchronous code, since the flow of execution is non-linear, it can sometimes be like trying to put together a puzzle. You might not see errors where you would expect them.
2. Learning curve
If you are used to synchronous programming this requires a completely different mindset. It can be challenging to understand how async/await
works and work with tasks.
3. Not ideal for CPU-bound tasks
Async programming works wonders for I/O-bound tasks (such as network calls or reading files), but if your task is CPU intensive, you may not benefit from much of a performance gain.
Async programming: Use cases & success stories
Asynchronous programming is leaving a remarkable footprint, particularly with the help of Python’s asyncio module
. Here are some of the use cases of Asynchronous programming along with real-world examples of companies that took maximum advantage of this approach:
1. Web servers
Web servers built using asynchronous frameworks like FastAPI or Sanic can easily handle thousands of requests concurrently without any issues. They manage and process multiple simultaneous connections, ensuring efficient and reliable access to web applications.
Example: Uber (a giant transport company) faced issues while handling thousands of requests per second and maintaining low response times. By using asynchronous programming, Uber optimized server resources and efficiently managed ride offers, driver locations, and real-time events, even during peak traffic.
2. Network applications
Whether its writing a chat server, game server or even some web scraper? You rely on async programming. It is a fantastic tool when it comes to juggling different network connections at the same time.
Example: Slack (a popular communication tool) uses async programming to manage its real-time messaging features. Along with that, Slack needs to keep thousands of teams in sync with each other live, they use asynchronous networking to run their app smoothly.
3. Database queries
If your app manages numerous queries through a database, you can utilize async programming that keeps those operations from blocking and leaves more resources for handling users.
Example: Airbnb (a vacation rental company) struggled with slow response times and unresponsive interfaces due to synchronous processing. By adopting asynchronous programming, the app efficiently fetches data in the background while keeping the user interface responsive.
4. Microservices architecture
Microservices, especially when services are interacting via the network need to communicate synchronously less frequently and that is where asynchronous frameworks come perfectly into the picture.
Example: Netflix (Yes, again) encountered performance challenges managing thousands of microservices for data and streaming. By adopting asynchronous programming, Netflix ensured efficient service communication and a seamless user experience.
Let’s move to the popular Python async frameworks that help manage multiple tasks efficiently and improve application performance.
Popular Python async frameworks
Have a look at some of the well-known Python asynchronous frameworks along with their respective examples of some giant companies:
1. FastAPI
Let’s explore FastAPI, a rapidly growing framework today used by many expert Python developers to build modern web APIs. It is lightning-fast and allows you to write your higher-level logic as async out of the box. It has automatic docs generation and easy async support, so it´s loved by most for microservices and API development.
Example: Microsoft faced challenges with handling a high volume of API requests efficiently. To address this, they adopted FastAPI with asynchronous support. This choice enables Microsoft to manage thousands of queries simultaneously, improving performance for backend services and data-processing tools.
2. Sanic
One more web framework that can effectively use the asynchronous features of Python is Sanic. It is designed for speed and uses an async programming style to handle multiple requests with lower overhead.
Example: Postmates (famous Logistics company) had a hard time with handling multiple customer requests simultaneously, such as order tracking and restaurant listings. To overcome this, they implemented Sanic with asynchronous programming. This solution allows Postmates to manage numerous requests at once, providing seamless user experiences and real-time delivery updates.
3. Aiohttp
Want a library for async HTTP requests? Aiohttp is the way to go. Aiohttp is an async networking library for Python and fits great with web scraping, APIs, and building a web server.
Example: Reddit uses Aiohttp to manage asynchronous HTTP requests between their backend systems. Using Aiohttp enables Reddit to accept thousands of requests per second while ensuring that browsing posts, submitting content, or interacting with each other will seamlessly continue during peak activity hours.
Want to keep going with Python? Explore our must-read blogs for more Python insights
-
Ultimate Guide to Python Frameworks for Scalable Microservices
-
Microservices Architecture with Python: Building Scalable Systems
Conclusion:
Implementing asynchronous programming with Python and the asyncio module alongside coroutines makes it a real ease to work on I/O-bound tasks. Whether you are writing web servers, handling database queries, or creating chat apps, using async programming is an excellent choice for non-blocking scalable solutions. Therefore if you are building a modern application and don't want every user to stare at your app while something is being made, then all those programming should be async-based.
Need help getting started? Now it is time to hire offshore Python developers who are well versed in the async world. They will have a much faster project turnover rate! Their knowledge will allow you to concentrate on implementation, while at the same time, your app is optimized for multithreaded execution ensuring that it achieves much-need enhanced performance.
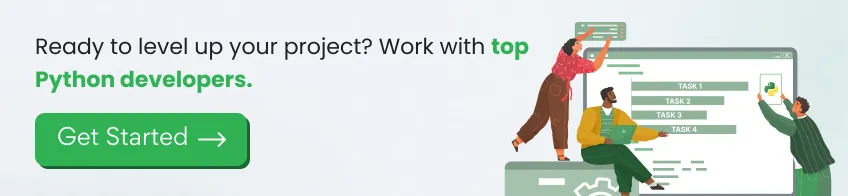